Post by alimularefin63 on Jun 9, 2024 11:28:17 GMT
In programming, understanding different data types and their conversions is crucial for efficient and accurate computations. Two commonly used data types are `decimal` and `double`. These types are integral to handling numerical data, each serving specific purposes depending on the required precision and range.
What Are Decimal and Double?
Decimal
The `decimal` data type is a 128-bit data type that offers a high level of precision AZB Directory and is primarily used in financial and monetary calculations where rounding errors can lead to significant issues. Decimals can represent a larger range of values with a high degree of accuracy, especially when dealing with fractions.
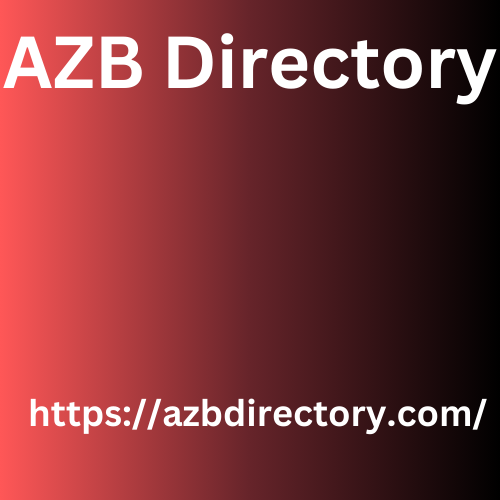
Double
The `double` data type, short for "double-precision floating-point," is a 64-bit data type used for storing large numbers and supporting a wide range of values. While it offers less precision compared to `decimal`, it is faster and consumes less memory. `Double` is commonly used in scientific computations where large dynamic ranges are more important than absolute precision.
Differences Between Decimal and Double
Precision
One of the primary differences between `decimal` and `double` is precision. Decimals are more precise and can handle more significant digits accurately, making them suitable for applications like financial calculations. Doubles, however, offer around 15-17 significant decimal digits, which might lead to precision loss in some calculations.
Performance
Doubles are faster to process because they are less precise and consume less memory (64-bit compared to 128-bit for decimals). This performance boost makes doubles ideal for applications requiring extensive numerical computations, such as scientific simulations or graphics processing.
Range
Doubles have a much larger range than decimals. While a `decimal` can represent numbers from approximately ±1.0 × 10^-28 to ±7.9 × 10^28, a `double` can represent numbers from approximately ±5.0 × 10^-324 to ±1.7 × 10^308. This makes doubles suitable for applications needing a wide range of values, even at the cost of some precision.
Why Convert Decimal to Double?
Performance Considerations
In some applications, the performance overhead associated with using `decimal` can be significant. Converting to `double` can improve the speed of numerical computations. For instance, in real-time systems or high-frequency trading platforms, the processing speed is critical, and using `double` can provide a performance boost.
Memory Efficiency
In scenarios where memory usage is a constraint, such as in embedded systems or large-scale data processing, using `double` instead of `decimal` can reduce memory footprint, allowing for more efficient data handling and storage.
How to Convert Decimal to Double
Explicit Conversion
In most programming languages, converting from `decimal` to `double` requires an explicit cast. Here’s an example in C:
```csharp
decimal decValue = 123.456M;
double dblValue = (double)decValue;
```
In this example, the `decimal` value `decValue` is explicitly cast to a `double` value `dblValue`.
Potential Issues
While converting from `decimal` to `double`, it’s essential to be aware of potential precision loss. Since `double` is less precise, some decimal values might not be represented accurately. It’s crucial to assess whether the precision loss is acceptable for your application before proceeding with the conversion.
Practical Applications
Financial Calculations
In financial applications, it’s common to use `decimal` for storing and manipulating monetary values due to the high precision required. However, during performance-critical operations, such as bulk data analysis or reporting, converting to `double` might be necessary to meet performance requirements.
Scientific Computations
In scientific and engineering applications, `double` is often the default choice due to its wide range and adequate precision for most calculations. However, in scenarios demanding even higher precision for specific computations, `decimal` might be used, with conversions to `double` as needed for performance optimization.
Conclusion
Understanding the differences between `decimal` and `double`, and knowing when and how to convert between these data types, is crucial for effective programming. Each data type has its strengths and use cases, and the choice between them should be guided by the specific requirements of your application in terms of precision, performance, and memory usage. By making informed decisions about data type conversions, you can optimize both the accuracy and efficiency of your software.